In this post, I demonstrate how to link Web App HTML pages together and pass parameters to the Web App HTML pages using Google Apps Script.
How to Video:
Video Notes:
- Legacy Apps Script Editor Used in Video
- Apps Script (Script Editor) is now located under tab ‘Extensions’ instead of ‘Tools’ on Google Sheets
- For Further Details on Deploying a Web App Click Here
Code in Video:
function doGet(e) {
if (!e.parameter.page)
{
var htmlOutput = HtmlService.createTemplateFromFile('Index');
htmlOutput.message = '';
return htmlOutput.evaluate();
}
else if(e.parameter['page'] == 'Link 1')
{
Logger.log(JSON.stringify(e));
var htmlOutput = HtmlService.createTemplateFromFile('Link 1');
htmlOutput.firstname = e.parameter['firstname'];
htmlOutput.lastname = e.parameter['lastname'];
return htmlOutput.evaluate();
}
else if(e.parameter['page'] == 'Link 2')
{
var htmlOutput = HtmlService.createTemplateFromFile('Link 2');
htmlOutput.firstname = e.parameter['firstname'];
htmlOutput.lastname = e.parameter['lastname'];
return htmlOutput.evaluate();
}
else if(e.parameter['page'] == 'Index')
{
var htmlOutput = HtmlService.createTemplateFromFile('Index');
htmlOutput.message = e.parameter['message'];
return htmlOutput.evaluate();
}
}
function getUrl() {
var url = ScriptApp.getService().getUrl();
return url;
}
<!DOCTYPE html>
<html>
<head>
<base target="_top">
<script>
function Link1()
{
var firstname = document.getElementById("firstname").value;
var lastname = document.getElementById("lastname").value;
var url = document.getElementById("url").value;
var link = document.createElement('a');
link.href = url+"?firstname="+firstname+"&lastname="+lastname+"&page=Link 1";
link.id = 'linkURL';
document.body.appendChild(link);
document.getElementById("linkURL").click();
}
function Link2()
{
var firstname = document.getElementById("firstname").value;
var lastname = document.getElementById("lastname").value;
var url = document.getElementById("url").value;
var link = document.createElement('a');
link.href = url+"?firstname="+firstname+"&lastname="+lastname+"&page=Link 2";
link.id = 'linkURL';
document.body.appendChild(link);
document.getElementById("linkURL").click();
}
</script>
</head>
<body>
<h1>Main Menu</h1>
<input type="button" value="Link 1" onclick="Link1()" /><br>
<input type="button" value="Link 2" onclick="Link2()" /><br><br>
<label>First Name</label><br>
<input type="text" id="firstname" /><br>
<label> Last Name</label><br>
<input type="text" id="lastname" /><br><br>
<span><?= message ?></span>
<?var url = getUrl();?><input type="hidden" value="<?= url ?>" id="url" />
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<base target="_top">
<script>
function Back()
{
var url = document.getElementById("url").value;
var link = document.createElement('a');
link.href = url+"?page=Index&message=Back From Link 1";
link.id = 'linkURL';
document.body.appendChild(link);
document.getElementById("linkURL").click();
}
</script>
</head>
<body>
<h1>Link 1</h1>
<input type="button" value="Back to Main Menu" onclick="Back()" /><br>
<span>FIRST NAME: <?= firstname ?></span><br>
<span>LAST NAME: <?= lastname ?></span><br>
<?var url = getUrl();?><input type="hidden" value="<?= url ?>" id="url" />
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<base target="_top">
<script>
function Back()
{
var url = document.getElementById("url").value;
var link = document.createElement('a');
link.href = url+"?page=Index&message=Back From Link 2";
link.id = 'linkURL';
document.body.appendChild(link);
document.getElementById("linkURL").click();
}
</script>
</head>
<body>
<h1>Link 2</h1>
<input type="button" value="Back to Main Menu" onclick="Back()" /><br>
<span>FIRST NAME: <?= firstname ?></span><br>
<span>LAST NAME: <?= lastname ?></span><br>
<?var url = getUrl();?><input type="hidden" value="<?= url ?>" id="url" />
</body>
</html>
Related Posts
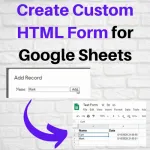
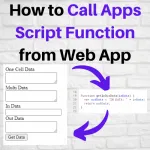
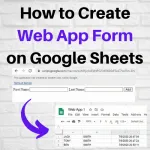
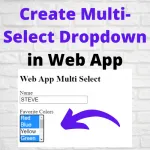
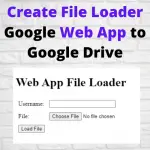
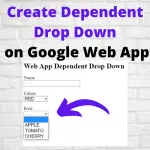